Python Quizzes
Check your Python learning progress and take your skills to the next level with Real Python’s interactive quizzes.
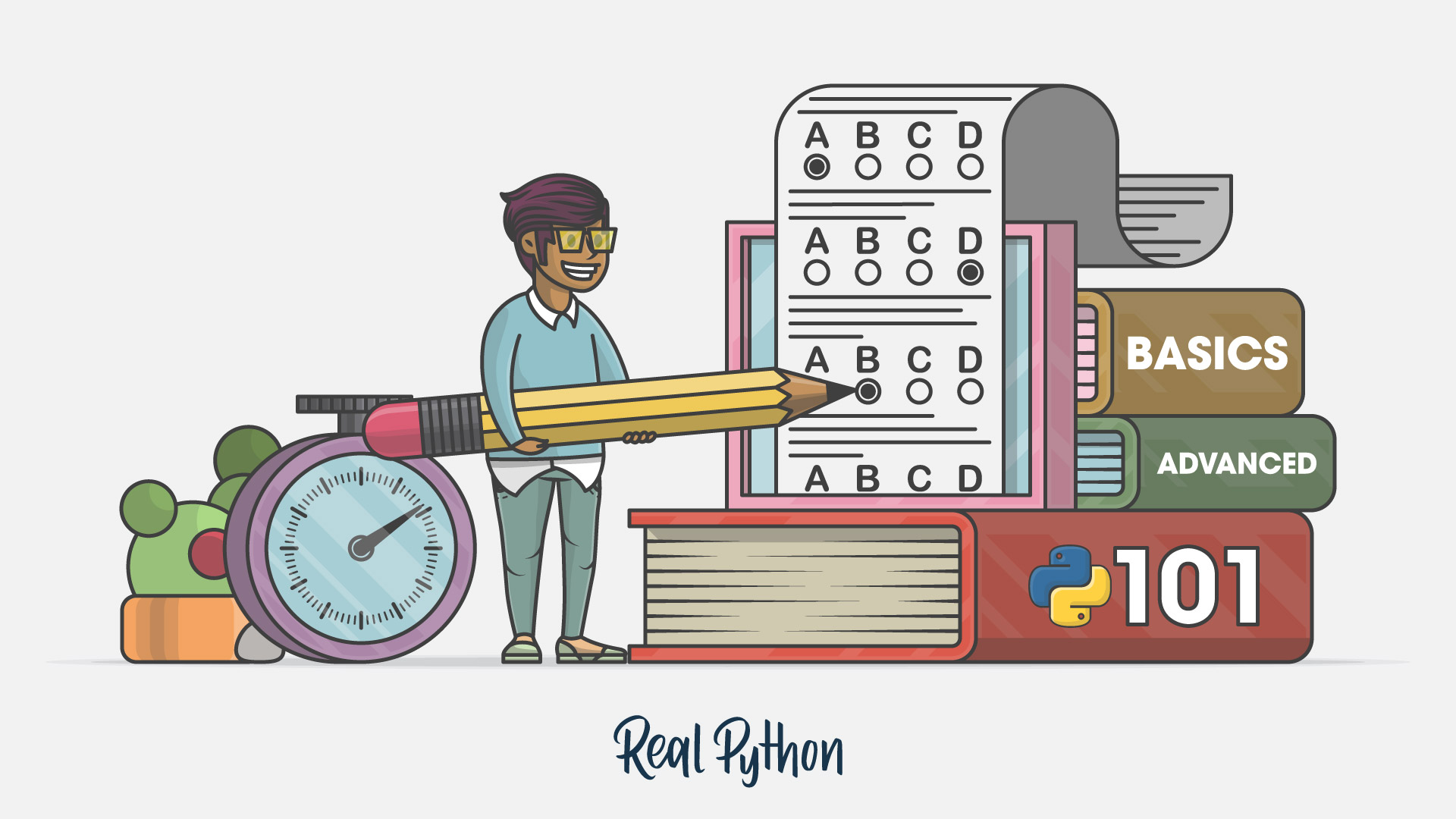
We created these online Python quizzes as a fun way for you to check your learning progress and to test your skills.
Each quiz takes you through a series of questions. Some of them are multiple choice, some will ask you to type in an answer, and some will require you to write actual Python code. As you make your way through each quiz, it keeps score of which questions you answered correctly.
At the end of each quiz you receive a grade based on your result. If you don’t score 100% on your first try—don’t fret! These quizzes are meant to challenge you and it’s expected that you go through them several times, improving your score with each run.
Browse Python Quizzes: Ready to test your Python skills? Pick a quiz below and jump right in, or search and filter quizzes by topic and skill level.
Interactive Quiz
A Guide to Modern Python String Formatting Tools
You can take this quiz to test your understanding of modern tools for string formatting in Python. These tools include f-strings and the .format() method.
Interactive Quiz
A Practical Introduction to Web Scraping in Python
In this quiz, you'll test your understanding of web scraping in Python. Web scraping is a powerful tool for data collection and analysis. By working through this quiz, you'll revisit how to parse website data using string methods, regular expressions, and HTML parsers, as well as how to interact with forms and other website components.
Interactive Quiz
Asynchronous Iterators and Iterables in Python
Take this quiz to test your understanding of how to create and use Python async iterators and iterables in the context of asynchronous code.
Interactive Quiz
Async IO in Python: A Complete Walkthrough
In this quiz, you'll test your understanding of async IO in Python. With this knowledge, you'll be able to understand the language-agnostic paradigm of asynchronous IO, use the async/await keywords to define coroutines, and use the asyncio package to run and manage coroutines.
Interactive Quiz
Basic Data Types in Python: A Quick Exploration
Take this quiz to test your understanding of the basic data types that are built into Python, like numbers, strings, bytes, and Booleans.
Interactive Quiz
Basic Input and Output in Python
In this quiz, you'll test your understanding of Python's built-in functions for user interaction, namely input() and print(). These functions allow you to capture user input from the keyboard and display output to the console, respectively.
Interactive Quiz
Beautiful Soup: Build a Web Scraper With Python
In this quiz, you'll test your understanding of web scraping using Python. By working through this quiz, you'll revisit how to inspect the HTML structure of a target site, decipher data encoded in URLs, and use Requests and Beautiful Soup for scraping and parsing data.
Interactive Quiz
Beautiful Soup: Build a Web Scraper With Python
In this quiz, you'll revisit the main steps of the web scraping process. You'll learn how to write a script that uses Python's Requests library to scrape data from a website. You'll also use Beautiful Soup to extract the specific pieces of information that you're interested in.
Interactive Quiz
Build a Blog Using Django, GraphQL, and Vue
In this quiz, you'll test your understanding of building a Django blog back end and a Vue front end, using GraphQL to communicate between them. This will help you decouple your back end and front end, handle data persistence in the API, and display the data in a single-page app (SPA).
Interactive Quiz
Build a Guitar Synthesizer
In this quiz, you'll test your understanding of what it takes to build a guitar synthesizer in Python. By working through this quiz, you'll revisit a few key concepts from music theory and sound synthesis.
Interactive Quiz
Build an LLM RAG Chatbot With LangChain
In this quiz, you'll test your understanding of building a retrieval-augmented generation (RAG) chatbot using LangChain and Neo4j. This knowledge will allow you to create custom chatbots that can retrieve and generate contextually relevant responses based on both structured and unstructured data.
Interactive Quiz
Build Command-Line Interfaces With Python's argparse
In this quiz, you'll test your understanding of creating command-line interfaces (CLIs) in Python using the argparse module. This knowledge is essential for creating user-friendly command-line apps, which are common in development, data science, and systems administration.
Interactive Quiz
Choosing the Best Font for Programming
In this quiz, you'll test your understanding of how to choose the best font for your daily programming. You'll get questions about the technicalities and features to consider when choosing a programming font and refresh your knowledge about how to spot a high-quality coding font.
Interactive Quiz
Context Managers and Python's with Statement
In this quiz, you'll assess your understanding of the Python with statement and context managers. By mastering these concepts, you'll be able to write more expressive code and manage system resources more effectively, avoiding resource leaks and ensuring proper setup and teardown of external resources.
Interactive Quiz
Creating Great README Files for Your Python Projects
Take this quiz to test your understanding of how a great README file can make your Python project stand out and how to create your own README files.
Interactive Quiz
Data Classes in Python
In this quiz, you'll test your understanding of Python data classes. Data classes, a feature introduced in Python 3.7, are a type of class mainly used for storing data. They come with basic functionality already implemented, such as instance initialization, printing, and comparison.
Interactive Quiz
Decorators
In this quiz, you'll revisit the foundational concepts of what Python decorators are and how to create and use them.
Interactive Quiz
Defining Main Functions in Python
In this quiz, you'll test your understanding of the Python main() function and the special __name__ variable. With this knowledge, you'll be able to understand the best practices for defining main() in Python.
Interactive Quiz
Defining Your Own Python Function
In this quiz, you'll test your understanding of how to define your own Python functions. You'll revisit both the basics and more complex syntax, such as args and kwargs, to sharpen your knowledge of function definitions in Python.
Interactive Quiz
Dictionaries in Python
In this quiz, you'll test your understanding of Python's dict data type. By working through this quiz, you'll revisit how to create and manipulate dictionaries, how to use Python's operators and built-in functions with them, and how they're implemented for efficient data retrieval.
Interactive Quiz
Documenting Python Code: A Complete Guide
In this quiz, you'll test your understanding of documenting Python code. With this knowledge, you'll be able to effectively document your Python scripts and projects, making them more understandable and maintainable.
Interactive Quiz
Effective Testing with Pytest
In this quiz, you'll test your understanding of pytest, a Python testing tool. With this knowledge, you'll be able to write more efficient and effective tests, ensuring your code behaves as expected.
Interactive Quiz
Expression vs Statement in Python: What's the Difference?
In this quiz, you'll test your understanding of Python expressions vs statements. Knowing the difference between these two is crucial for writing efficient and readable Python code.
Interactive Quiz
Format Floats Within F-Strings
In this quiz, you'll test your understanding of how to format floats within f-strings in Python. This knowledge will let you control the precision and appearance of floating-point numbers when you incorporate them into formatted strings.
Interactive Quiz
Functional Programming in Python: When and How to Use It
In this quiz, you'll test your understanding of functional programming in Python. You'll revisit concepts such as functions being first-class citizens in Python, the use of the lambda keyword, and the implementation of functional code using map(), filter(), and reduce().
Interactive Quiz
Generate Images With DALL·E and the OpenAI API
In this quiz, you'll test your understanding of generating images with DALL·E by OpenAI using Python. You'll revisit concepts such as using the OpenAI Python library, making API calls for image generation, creating images from text prompts, and converting Base64 strings to PNG image files.
Interactive Quiz
Get Started With Django: Build a Portfolio App
In this quiz, you'll test your understanding of Django, a fully featured Python web framework. By working through this quiz, you'll revisit the steps to create a fully functioning web application and learn about some of Django's most important features.
Interactive Quiz
Getters and Setters: Manage Attributes in Python
In this quiz, you'll test your understanding of Python's getter and setter methods, as well as properties. You'll learn when to use these tools and how they can help maintain encapsulation in your classes.
Interactive Quiz
Getting Started With Async Features in Python
In this quiz, you'll test your understanding of asynchronous programming in Python. You'll revisit the concepts of synchronous and asynchronous programs, and why you might want to write an asynchronous program. You'll also test your knowledge on how to use Python async features.
Interactive Quiz
Getting Started With Python IDLE
In this quiz, you'll test your understanding of Python IDLE, the default integrated development environment (IDE) that comes bundled with every Python installation. You'll revisit how to interact with Python directly in IDLE, edit and execute Python files.
Interactive Quiz
Getting Started With Testing in Python
In this quiz, you'll test your understanding of Python testing. With this knowledge, you'll be able to create basic tests, execute them, and find bugs before your users do.
Interactive Quiz
GitHub Actions for Python
In this quiz, you'll test your understanding of GitHub Actions for Python. By working through this quiz, you'll revisit how to use GitHub Actions and workflows to automate linting, testing, and deployment of a Python project.
Interactive Quiz
How Do You Choose Python Function Names?
In this quiz, you'll test your understanding of how to choose Python function names. By working through this quiz, you'll revisit the rules and conventions for naming Python functions and why they're important for writing Pythonic code.
Interactive Quiz
How to Check if a Python String Contains a Substring
In this quiz, you'll check your understanding of the best way to check whether a Python string contains a substring. You'll also revisit idiomatic ways to inspect the substring further, match substrings with conditions using regular expressions, and search for substrings in pandas.
Interactive Quiz
How to Create Pivot Tables With pandas
This quiz is designed to push your knowledge of pivot tables a little bit further. You won't find all the answers by reading the tutorial, so you'll need to do some investigating on your own. By finding all the answers, you're sure to learn some other interesting things along the way.
Interactive Quiz
How to Deal With Missing Data in Polars
This quiz will test your knowledge of working with null data in Polars. You won't find all the answers in the tutorial, so you'll need to do some extra investigating. By finding all the answers, you're sure to learn some interesting things along the way.
Interactive Quiz
How to Exit Loops Early With the Python Break Keyword
In this quiz, you'll test your understanding of the Python break statement. This keyword allows you to exit a loop prematurely, transferring control to the code that follows the loop.
Interactive Quiz
How to Flatten a List of Lists in Python
In this quiz, you'll test your understanding of how to flatten a list in Python. Flattening a list involves converting a multidimensional list, such as a matrix, into a one-dimensional list. This is a common operation when working with data stored as nested lists.
Interactive Quiz
How to Join Strings in Python
Test your understanding of Python's .join() string method for combining strings, handling edge cases, and optimizing performance.
Interactive Quiz
How to Manage Python Projects With pyproject.toml
In this quiz, you'll test your understanding of Python's pyproject.toml file, which simplifies Python project configuration by unifying package setup, managing dependencies, and streamlining builds.
Interactive Quiz
How to Remove Items From Lists in Python
In this quiz, you'll test your understanding of removing items from lists in Python. This is a fundamental skill in Python programming, and mastering it will enable you to manipulate lists effectively.
Interactive Quiz
How to Reset a pandas DataFrame Index
This quiz will challenge your knowledge of resetting indexes in pandas DataFrames. You won't find all the answers in the tutorial, so you'll need to do some extra investigating. By finding all the answers, you're sure to learn some interesting things along the way.
Interactive Quiz
How to Run Your Python Scripts
One of the most important skills you need to build as a Python developer is to be able to run Python scripts and code. Test your understanding on how good you are with running your code.
Interactive Quiz
How to Split a String in Python
In this quiz, you'll test your understanding of Python's .split() method. This method is useful for text-processing and data parsing tasks, allowing you to divide a string into a list of substrings based on a specified delimiter.
Interactive Quiz
How to Strip Characters From a Python String
In this quiz, you'll test your understanding of Python's .strip(), .lstrip(), and .rstrip() methods, as well as .removeprefix() and .removesuffix(). These methods are useful for tasks like cleaning user input, standardizing filenames, and preparing data for storage.
Interactive Quiz
How to Use Conditional Expressions With NumPy where()
This quiz aims to test your understanding of the np.where() function. You won't find all the answers in the tutorial, so you'll need to do additional research. It's recommended that you make sure you can do all the exercises in the tutorial before tackling this quiz. Enjoy!
Interactive Quiz
How to Use Generators and yield in Python
In this quiz, you'll test your understanding of Python generators and the yield statement. With this knowledge, you'll be able to work with large datasets in a more Pythonic fashion, create generator functions and expressions, and build data pipelines.
Interactive Quiz
How to Use sorted() and .sort() in Python
In this quiz, you'll test your understanding of sorting in Python using sorted() and .sort(). You'll revisit how to sort various types of data in different data structures, customize the order, and work with two different ways of sorting in Python.
Interactive Quiz
How to Work With Polars LazyFrames
This quiz will challenge your knowledge of working with Polars LazyFrames. You won't find all the answers in the tutorial, so you'll need to do some extra investigating. By finding all the answers, you're sure to learn some interesting things along the way.
Interactive Quiz
How to Write Beautiful Python Code With PEP 8
In this quiz, you'll test your understanding of PEP 8, the Python Enhancement Proposal that provides guidelines and best practices on how to write Python code. By working through this quiz, you'll revisit the key guidelines laid out in PEP 8 and how to set up your development environment to write PEP 8 compliant Python code.
Interactive Quiz
HTTP Requests With the "requests" Library
Test your understanding of the Python "requests" library for making HTTP requests and interacting with web services.
Interactive Quiz
Hugging Face Transformers
In this quiz, you'll test your understanding of the Hugging Face Transformers library. This library is a popular choice for working with transformer models in natural language processing tasks, computer vision, and other machine learning applications.
Interactive Quiz
Implementing an Interface in Python
In this quiz, you'll test your understanding of Python interfaces and their role in software engineering. You'll learn how interfaces can help manage complexity in a growing application and how to implement them in Python.
Interactive Quiz
Inheritance and Composition: A Python OOP Guide
In this quiz, you'll test your understanding of inheritance and composition in Python. These are two major concepts in object-oriented programming that help model the relationship between two classes. By working through this quiz, you'll revisit how to use inheritance and composition in Python, model class hierarchies, and use multiple inheritance.
Interactive Quiz
Install and Execute Python Applications Using pipx
In this quiz, you'll test your understanding of how Python apps are run from isolated virtual environments using the pipx tool. With this knowledge, you'll be able to safely run Python apps that are installed globally in your operating system.
Interactive Quiz
Interacting With Python
In this quiz, you'll test your understanding of the different ways of interacting with Python. By working through this quiz, you'll revisit key concepts related to Python interaction in interactive mode using the REPL, through Python script files, and within IDEs and code editors.
Interactive Quiz
Introducing DuckDB
This quiz will challenge your knowledge of working with DuckDB. You won't find all the answers in the tutorial, so you'll need to do some extra investigation. By finding all the answers, you're sure to learn some interesting things along the way.
Interactive Quiz
Iterators and Iterables in Python: Run Efficient Iterations
In this quiz, you'll test your understanding of Python's iterators and iterables. By working through this quiz, you'll revisit how to create and work with iterators and iterables, the differences between them, and review how to use generator functions.
Interactive Quiz
LangGraph: Build Stateful AI Agents in Python
Take this quiz to test your understanding of LangGraph, a Python library designed for stateful, cyclic, and multi-actor Large Language Model (LLM) applications. By working through this quiz, you'll revisit how to build LLM workflows and agents in LangGraph.
Interactive Quiz
Linear Regression in Python
In this quiz, you'll test your knowledge of linear regression in Python. Linear regression is one of the fundamental statistical and machine learning techniques, and Python is a popular choice for machine learning.
Interactive Quiz
Lists vs Tuples in Python
Challenge yourself with this quiz to evaluate and deepen your understanding of Python lists and tuples. You'll explore key concepts, such as how to create, access, and manipulate these data types, while also learning best practices for using them efficiently in your code.
Interactive Quiz
Logging in Python
In this quiz, you'll test your understanding of Python's logging module. With this knowledge, you'll be able to add logging to your applications, which can help you debug errors and analyze performance.
Interactive Quiz
Managing Multiple Python Versions With pyenv
In this quiz, you'll test your understanding of how to use pyenv to manage multiple versions of Python. Pyenv allows you to try out new Python features without disrupting your development environment, and quickly switch between different Python versions.
Interactive Quiz
Managing Python Projects With uv: An All-in-One Solution
In this quiz, you'll test your understanding of the uv tool, a high-speed package and project manager for Python.
Interactive Quiz
Model-View-Controller (MVC) in Python Web Apps: Explained With Lego
In this quiz, you'll test your understanding of the Model-View-Controller (MVC) design pattern, a fundamental concept in many Python web frameworks. By working through this quiz, you'll revisit the concepts of Models, Views, and Controllers, and how they relate to concrete web development examples.
Interactive Quiz
Namespaces and Scope in Python
In this quiz, you'll test your understanding of Python namespaces and variable scope. These concepts are crucial for organizing the symbolic names assigned to objects in a Python program and ensuring they don't interfere with one another.
Interactive Quiz
Namespaces in Python
In this quiz, you'll test your understanding of Python namespaces. These concepts are crucial for organizing the symbolic names assigned to objects in a Python program and ensuring they don't interfere with one another.
Interactive Quiz
NumPy Practical Examples: Useful Techniques
This quiz will test your understanding of working with NumPy arrays. You won't find all the answers in the tutorial, so you'll need to do some extra investigating. By finding all the answers, you're sure to learn some interesting things along the way.
Interactive Quiz
Object-Oriented Programming (OOP) in Python
Object-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects.
Interactive Quiz
Practical Prompt Engineering
In this quiz, you'll test your understanding of prompt engineering techniques with large language models (LLMs) like GPT-3.5 and GPT-4. You'll revisit how to work with OpenAI's API, apply prompt engineering techniques to practical examples, and use various strategies to improve your results.
Interactive Quiz
Primer on Jinja Templating
In this quiz, you'll test your understanding of Jinja templating. Jinja is a powerful tool for building rich templates in Python web applications, and it can also be used to create text files with programmatic content.
Interactive Quiz
Pydantic: Simplifying Data Validation in Python
In this quiz, you'll test your understanding of Pydantic, a powerful data validation library for Python. You'll revisit concepts such as working with data schemas, writing custom validators, validating function arguments, and managing settings with pydantic-settings.
Interactive Quiz
Python 3.13: Cool New Features for You to Try
In this quiz, you'll test your understanding of the new features introduced in Python 3.13. By working through this quiz, you'll review the key updates and improvements in this version of Python.
Interactive Quiz
Python 3.13: Free Threading and a JIT Compiler
In this quiz, you'll test your understanding of the new features in Python 3.13. You'll revisit how to compile a custom Python build, disable the Global Interpreter Lock (GIL), enable the Just-In-Time (JIT) compiler, and more.
Interactive Quiz
Python and REST APIs: Interacting With Web Services
In this quiz, you'll test your understanding of REST APIs and how to interact with them using Python. With this knowledge, you'll be able to retrieve, parse, update, and manipulate data provided by any RESTful API that you're interested in.
Interactive Quiz
Python args and kwargs: Demystified
In this quiz, you'll test your understanding of how to use *args and **kwargs in Python. With this knowledge, you'll be able to add more flexibility to your functions.
Interactive Quiz
Python Basics: Building Systems With Classes
You can use classes to build complex systems in Python. By composing classes, inheriting from other classes, and overriding class behavior, you'll harness the power of object-oriented programming (OOP).
Interactive Quiz
Python Basics: Code Your First Python Program
With Python installed, you're ready ready to start coding! It's time to write your first Python program.
Interactive Quiz
Python Basics: Conditional Logic and Control Flow
With conditional logic, you can write programs that perform different actions based on different conditions. Paired with functions and loops, conditional logic allows you to write complex programs that can handle many different situations.
Interactive Quiz
Python Basics: Dictionaries
Python dictionaries, like lists and tuples, store a collection of objects. However, instead of storing objects in a sequence, dictionaries hold information in pairs of data called key-value pairs.
Interactive Quiz
Python Basics: File System Operations
The pathlib module allows you to carry out file path operations with Python. These operations include creating, iterating over, searching for, moving, and deleting files and folders.
Interactive Quiz
Python Basics: Finding and Fixing Code Bugs
Logic errors occur when an otherwise valid program doesn't do what was intended. They cause unexpected behaviors called bugs. Removing bugs is called debugging, and a debugger is a tool that helps you hunt down bugs and understand why they're happening.
Interactive Quiz
Python Basics: Functions and Loops
Functions break code into smaller chunks and are great for defining actions that a program will execute several times throughout your code. Instead of writing the same code each time the program needs to perform the same task, just call the function!
Interactive Quiz
Python Basics: Installing Packages With pip Quiz
Even though Python is famous for coming with batteries included, you'll still find yourself in need of a third-party library from time to time. You can install such packages with pip.
Interactive Quiz
Python Basics: Lists and Tuples
Lists and tuples are important sequence data structures in Python. Lists are mutable, while tuples are immutable. Combining your knowledge of control flow and loops with these sequences allows you to build complex logic.
Interactive Quiz
Python Basics: Modules and Packages
You can build an application by putting related code into separate files called modules. Then, you can use the import statement to use modules in another file.
Interactive Quiz
Python Basics: Numbers and Math
You don't need to be a math whiz to program well, but numbers are an integral part of any programming language. Python is no exception.
Interactive Quiz
Python Basics: Object-Oriented Programming
OOP, or object-oriented programming, is a method of structuring a program by bundling related properties and behaviors into individual objects. In this quiz, you'll test your understanding of OOP.
Interactive Quiz
Python Basics: Reading and Writing Files Quiz
By reading and writing files, you can move data back and forth between your Python programs and external software. The CSV file format is particularly useful, as it's one of the most widely supported file formats for transferring tabular data.
Interactive Quiz
Python Basics: Scopes
To fully understand functions and loops in Python, you need to be familiar with the issue of scope, which determines where a name is visible within your program.
Interactive Quiz
Python Basics: Strings and String Methods
Strings are a fundamental data type in Python. In simplified terms, strings are collections of text, and they show up in many contexts. For example, strings can come from user input, data read from a file, or messages sent by equipment talking over a network.
Interactive Quiz
Python Bytes
In this quiz, you'll test your understanding of Python bytes objects. By working through this quiz, you'll revisit the key concepts related to this low-level data type.
Interactive Quiz
Python Class Constructors: Control Your Object Instantiation
In this quiz, you'll test your understanding of class constructors in Python. By working through this quiz, you'll revisit the internal instantiation process, object initialization, and fine-tuning object creation.
Interactive Quiz
Python Classes - The Power of Object-Oriented Programming
In this quiz, you'll test your understanding of Python classes. With this knowledge, you'll be able to define reusable pieces of code that encapsulate data and behavior in a single entity, model real-world objects, and solve complex problems.
Interactive Quiz
Python Closures: Common Use Cases and Examples
In this quiz, you'll test your understanding of Python closures. Closures are a common feature in functional programming languages and are particularly popular in Python because they allow you to create function-based decorators.
Interactive Quiz
Python Code Quality: Best Practices and Tools
In this quiz, you'll test your understanding of Python code quality, tools, and best practices. By working through this quiz, you'll revisit the importance of producing high-quality Python code that's functional, readable, maintainable, efficient, and secure.
Interactive Quiz
Python Concurrency
In this quiz, you'll test your understanding of Python concurrency. You'll revisit the different forms of concurrency in Python, how to implement multi-threaded and asynchronous solutions for I/O-bound tasks, and how to achieve true parallelism for CPU-bound tasks.
Interactive Quiz
Python Conditional Statements
Test your understanding of Python conditional statements
Interactive Quiz
Python Dictionary Comprehensions: How and When to Use Them
In this quiz, you'll test your understanding of Python dictionary comprehensions. Dictionary comprehensions are a concise and quick way to create, transform, and filter dictionaries in Python, and can significantly enhance your code's conciseness and readability.
Interactive Quiz
Python Dictionary Iteration
Dictionaries are one of the most important and useful data structures in Python. Learning how to iterate through a Dictionary can help you solve a wide variety of programming problems in an efficient way. Test your understanding on how you can use them better!
Interactive Quiz
Python Exceptions: An Introduction
In this quiz, you'll test your understanding of Python exceptions. You'll cover the difference between syntax errors and exceptions and learn how to raise exceptions, make assertions, and use the try and except block.
Interactive Quiz
Python Folium: Create Web Maps From Your Data
Python’s Folium library gives you access to the mapping strengths of the Leaflet JavaScript library through a Python API. It allows you to create interactive geographic visualizations that you can share as a website.
Interactive Quiz
Python for Loops: The Pythonic Way
In this quiz, you'll test your understanding of Python's for loop. You'll revisit how to iterate over items in a data collection, how to use range() for a predefined number of iterations, and how to use enumerate() for index-based iteration.
Interactive Quiz
Python F-Strings
In this quiz, you'll test your knowledge of Python f-strings. With this knowledge, you'll be able to include all sorts of Python expressions inside your strings.
Interactive Quiz
Python GUI Programming With Tkinter
In this quiz, you'll test your understanding of Python GUI Programming With Tkinter, the de facto Python GUI framework. Check your knowledge of GUI programming concepts such as widgets, geometry managers, and event handlers.
Interactive Quiz
Python import: Advanced Techniques and Tips
In this quiz, you'll test your understanding of Python's import statement and how it works. You'll revisit your understanding of how to use modules and how to import modules dynamically at runtime.
Interactive Quiz
Python Installation and Setup
In this quiz, you'll test your understanding of how to install or update Python on your computer. With this knowledge, you'll be able to set up Python on various operating systems, including Windows, macOS, and Linux.
Interactive Quiz
Python Keywords: An Introduction
In this quiz, you'll test your understanding of Python keywords and soft keywords. These reserved words have specific functions and restrictions in Python, and understanding how to use them correctly is fundamental for building Python programs.
Interactive Quiz
Python Lambda Functions
Python lambdas are little, anonymous functions, subject to a more restrictive but more concise syntax than regular Python functions. Test your understanding on how you can use them better!
Interactive Quiz
Python Logging With the Loguru Library
Think you know Python logging? Take this quick Loguru quiz to test your knowledge of formatting, sinks, rotation, and more!
Interactive Quiz
Python Mappings
In this quiz, you'll test your understanding of the basic characteristics and operations of Python mappings. By working through this quiz, you'll revisit the key concepts and techniques of creating a custom mapping.
Interactive Quiz
Python Modules and Packages
In this quiz, you'll test your understanding of Python modules and packages, which are mechanisms that facilitate modular programming. Modular programming involves breaking a large programming task into smaller, more manageable subtasks or modules. This approach has several advantages, including simplicity, maintainability, and reusability.
Interactive Quiz
Python Name-Main Idiom
Test your knowledge of Python's if __name__ == "__main__" idiom by answering a series of questions! You've probably encountered the name-main idiom and might have even used it in your own scripts. But did you use it correctly?
Interactive Quiz
Python Operators and Expressions
Test your understanding of Python operators and expressions.
Interactive Quiz
Python Protocols: Leveraging Structural Subtyping
Take this quiz to test your understanding of how to create and use Python protocols while providing type hints for your functions, variables, classes, and methods.
Interactive Quiz
Python Raw Strings
In this quiz, you can practice your understanding of how to use raw string literals in Python. With this knowledge, you'll be able to write cleaner and more readable regular expressions, Windows file paths, and many other string literals that deal with escape character sequences.
Interactive Quiz
Python's Built-in Exceptions: A Walkthrough With Examples
In this quiz, you'll test your understanding of Python's built-in exceptions. With this knowledge, you'll be able to effectively identify and handle these exceptions when they appear. Additionally, you'll be more familiar with how to raise some of these exceptions in your code.
Interactive Quiz
Python's Built-in Functions: A Complete Exploration
Take this quiz to test your knowledge about the available built-in functions in Python. By taking this quiz, you'll deepen your understanding of how to use these functions and the common programming problems they cover, from mathematical computations to Python-specific features.
Interactive Quiz
Python's Bytearray
In this quiz, you'll test your understanding of Python's bytearray data type. By working through this quiz, you'll revisit the key concepts and uses of bytearray in Python.
Interactive Quiz
Python's enumerate()
Once you learn about for loops in Python, you know that using an index to access items in a sequence isn't very Pythonic. So what do you do when you need that index value? In this tutorial, you'll learn all about Python's built-in enumerate(), where it's used, and how you can emulate its behavior.
Interactive Quiz
Python Sequences: A Comprehensive Guide
In this quiz, you'll test your understanding of sequences in Python. You'll revisit the basic characteristics of a sequence, operations common to most sequences, special methods associated with sequences, and how to create user-defined mutable and immutable sequences.
Interactive Quiz
Python Set Comprehensions: How and When to Use Them
In this quiz, you'll test your understanding of Python set comprehensions. Set comprehensions are a concise and quick way to create, transform, and filter sets in Python. They can significantly enhance your code's conciseness and readability compared to using regular for loops to process your sets.
Interactive Quiz
Python Sets
In this quiz, you'll assess your understanding of Python's built-in set data type. You'll revisit the definition of unordered, unique, hashable collections, how to create and initialize sets, and key set operations.
Interactive Quiz
Python's Instance, Class, and Static Methods Demystified
In this quiz, you'll test your understanding of instance, class, and static methods in Python. By working through this quiz, you'll revisit the differences between these methods and how to use them effectively in your Python code.
Interactive Quiz
Python's Magic Methods: Leverage Their Power in Your Classes
In this quiz, you'll test your understanding of Python's magic methods. These special methods are fundamental to object-oriented programming in Python, allowing you to customize the behavior of your classes.
Interactive Quiz
Python's property(): Add Managed Attributes to Your Classes
In this quiz, you'll test your understanding of Python's property(). With this knowledge, you'll be able to create managed attributes in your classes, perform lazy attribute evaluation, provide computed attributes, and more.
Interactive Quiz
Python's raise: Effectively Raising Exceptions in Your Code
In this quiz, you'll test your understanding of how to raise exceptions in Python using the raise statement. This knowledge will help you handle errors and exceptional situations in your code, leading to more robust programs and higher-quality code.
Interactive Quiz
Python String Formatting: Available Tools and Their Features
You can take this quiz to test your understanding of the available tools for string formatting in Python, as well as their strengths and weaknesses. These tools include f-strings, the .format() method, and the modulo operator.
Interactive Quiz
Python Strings and Character Data
This quiz will test your understanding of Python's string data type and your knowledge about manipulating textual data with string objects. You'll cover the basics of creating strings using literals and the str() function, applying string methods, using operators and built-in functions, and more!
Interactive Quiz
Python's unittest: Writing Unit Tests for Your Code
In this quiz, you'll test your understanding of Python testing with the unittest framework from the standard library. With this knowledge, you'll be able to create basic tests, execute them, and find bugs before your users do.
Interactive Quiz
Python Textual: Build Beautiful UIs in the Terminal
In this quiz, you'll test your understanding of the Python Textual library. This library is used to create rich terminal applications and widgets. By working through this quiz, you'll reinforce your knowledge of Textual's key concepts and features.
Interactive Quiz
Python Threading
This is a quiz that will review topics covered in our An Intro To Threading tutorial.
Interactive Quiz
Python Thread Safety: Using a Lock and Other Techniques
In this quiz, you'll test your understanding of Python thread safety. You'll revisit the concepts of race conditions, locks, and other synchronization primitives in the threading module. By working through this quiz, you'll reinforce your knowledge about how to make your Python code thread-safe.
Interactive Quiz
Python time.sleep()
In this quiz, you'll revisit how to add time delays to your Python programs.
Interactive Quiz
Python Type Checking
In this quiz, you'll test your understanding of Python type checking. You'll revisit concepts such as type annotations, type hints, adding static types to code, running a static type checker, and enforcing types at runtime. This knowledge will help you develop your code more efficiently.
Interactive Quiz
Python Virtual Environments: A Primer
In this quiz, you'll test your understanding of Python virtual environments. With this knowledge, you'll be able to avoid dependency conflicts and help other developers reproduce your development environment.
Interactive Quiz
Python while Loops: Repeating Tasks Conditionally
In this quiz, you'll test your understanding of Python's while loop. This loop allows you to execute a block of code repeatedly as long as a given condition remains true. Understanding how to use while loops effectively is a crucial skill for any Python developer.
Interactive Quiz
Reading and Writing CSV Files in Python
This quiz will check your understanding of what a CSV file is and the different ways to read and write to them in Python.
Interactive Quiz
Reading and Writing Files in Python
A quiz used for testing the user's knowledge of the topics covered in the Reading and Writing Files in Python article.
Interactive Quiz
Reading and Writing WAV Files in Python
In this quiz, you can test your knowledge of handling WAV audio files in Python with the wave module. By applying what you've learned, you'll demonstrate your ability to synthesize sounds, analyze and visualize waveforms, create dynamic spectrograms, and enhance audio with special effects.
Interactive Quiz
Ruff: A Modern Python Linter
In this quiz, you'll test your understanding of Ruff, a modern linter for Python. By working through this quiz, you'll revisit why you'd want to use Ruff to check your Python code and how it automatically fixes errors, formats your code, and provides optional configurations to enhance your linting.
Interactive Quiz
Shallow vs Deep Copying of Python Objects
In this quiz, you'll test your understanding of Python's copy module, which provides tools for creating shallow and deep copies of objects. This knowledge is crucial for managing complex, mutable data structures safely and effectively.
Interactive Quiz
Single and Double Underscores in Python Names
In this quiz, you'll test your understanding of the use of single and double underscores in Python names. This knowledge will help you differentiate between public and non-public names, avoid name clashes, and write code that looks Pythonic and consistent.
Interactive Quiz
Socket Programming in Python
In this quiz, you'll test your understanding of Python sockets. With this knowledge, you'll be able to create your own client-server applications, handle multiple connections simultaneously, and send messages and data between endpoints.
Interactive Quiz
Split Your Dataset With scikit-learn's train_test_split()
In this quiz, you'll test your understanding of how to use the train_test_split() function from the scikit-learn library to split your dataset into subsets for unbiased evaluation in machine learning.
Interactive Quiz
String Interpolation in Python: Exploring Available Tools
Take this quiz to test your understanding of the available tools for string interpolation in Python, as well as their strengths and weaknesses. These tools include f-strings, the .format() method, and the modulo operator.
Interactive Quiz
Structural Pattern Matching
In this quiz, you'll test your understanding of structural pattern matching in Python. This powerful control flow construct, introduced in Python 3.10, offers concise and readable syntax while promoting a declarative code style.
Interactive Quiz
Supercharge Your Classes With Python super()
In this quiz, you'll test your understanding of inheritance and the super() function in Python. By working through this quiz, you'll revisit the concept of inheritance, multiple inheritance, and how the super() function works in both single and multiple inheritance scenarios.
Interactive Quiz
Syntactic Sugar: Why Python Is Sweet and Pythonic
You can take this quiz to test your understanding of Python's most common pieces of syntactic sugar and how they make your code more Pythonic and readable.
Interactive Quiz
The Python calendar Module
In this quiz, you'll test your understanding of the calendar module in Python. It'll evaluate your proficiency in manipulating, customizing, and displaying calendars directly within your terminal. By working through this quiz, you'll revisit the fundamental functions and methods provided by the calendar module.
Interactive Quiz
The Python print() Function
In this interactive quiz, you can revisit what you know about Python's print() function. You'll also get to quiz yourself about some of its lesser-known features.
Interactive Quiz
The Python return Statement
In this quiz, you can practice your understanding of how to use the Python return statement when writing functions. Additionally, you'll cover some good programming practices related to the use of return. With this knowledge, you'll be able to write readable, robust, and maintainable functions in Python.
Interactive Quiz
The Python Standard REPL: Try Out Code and Ideas Quickly
In this quiz, you'll test your understanding of the Python standard REPL. The Python REPL allows you to run Python code interactively, which is useful for testing new ideas, exploring libraries, refactoring and debugging code, and trying out examples.
Interactive Quiz
The Walrus Operator: Python's Assignment Expressions
In this quiz, you'll test your understanding of Python's walrus operator. This operator was introduced in Python 3.8, and understanding it can help you write more concise and efficient code.
Interactive Quiz
Understanding the Python Mock Object Library
In this quiz, you'll test your understanding of Python's unittest.mock library. With this knowledge, you'll be able to write robust tests, create mock objects, and ensure your code is reliable and efficient.
Interactive Quiz
Using and Creating Global Variables in Your Python Functions
In this quiz, you'll test your understanding of how to use global variables in Python functions. With this knowledge, you'll be able to share data across an entire program, modify and create global variables within functions, and understand when to avoid using global variables.
Interactive Quiz
Using Python's .__dict__ to Work With Attributes
In this quiz, you'll test your understanding of Python's .__dict__ attribute and its usage in classes, instances, and functions. Acting as a namespace, this attribute maps attribute names to their corresponding values and serves as a versatile tool for metaprogramming and debugging.
Interactive Quiz
Using Python's pip to Manage Your Projects' Dependencies
In this quiz, you'll test your understanding of Python's standard package manager, pip. You'll revisit the ideas behind pip, important commands, and how to install packages.
Interactive Quiz
Using .__repr__() vs .__str__() in Python
In this quiz, you'll test your understanding of Python's dunder repr and dunder str special methods. These methods allow you to control how a program displays an object, making your classes more readable and easier to debug and maintain.
Interactive Quiz
Variables in Python: Usage and Best Practices
In this quiz, you'll test your understanding of variables in Python. Variables are symbolic names that refer to objects or values stored in your computer's memory, and they're essential building blocks for any Python program.
Interactive Quiz
Web Automation With Python and Selenium
In this quiz, you'll test your understanding of using Selenium with Python for web automation. You'll revisit concepts like launching browsers, interacting with web elements, handling dynamic content, and implementing the Page Object Model (POM) design pattern.
Interactive Quiz
Web Scraping With Scrapy and MongoDB
In this quiz, you'll test your understanding of web scraping with Scrapy and MongoDB. You'll revisit how to set up a Scrapy project, build a functional web scraper, extract data from websites, store scraped data in MongoDB, and test and debug your Scrapy web scraper.
Interactive Quiz
What Are CRUD Operations?
In this quiz, you'll revisit the key concepts and techniques related to CRUD operations. These operations are fundamental to any system that interacts with a database, and understanding them is crucial for effective data management.
Interactive Quiz
What Is the __pycache__ Folder in Python?
In this quiz, you'll have the opportunity to test your knowledge of the __pycache__ folder, including when, where, and why Python creates these folders.
Interactive Quiz
What Is the Python Global Interpreter Lock (GIL)?
In this quiz, you'll test your understanding of the Python Global Interpreter Lock (GIL). The GIL behaves like a mutex that allows only one thread to hold the control of the Python interpreter. This has advantages, but can be a performance bottleneck in CPU-bound and multi-threaded code.
Interactive Quiz
What's Lazy Evaluation in Python?
In this quiz, you'll test your understanding of the differences between lazy and eager evaluation in Python. By working through this quiz, you'll revisit how Python optimizes memory use and computational overhead by deciding when to compute values.
Interactive Quiz
When to Use a List Comprehension in Python
In this quiz, you'll test your understanding of Python list comprehensions. You'll revisit how to rewrite loops as list comprehensions, how to choose between comprehensions and loops, and how to use conditional logic in your comprehensions.
Interactive Quiz
Working With JSON Data in Python
In this quiz, you'll test your understanding of working with JSON in Python. By working through this quiz, you'll revisit key concepts related to JSON data manipulation and handling in Python.
Interactive Quiz
Write Pythonic and Clean Code With namedtuple
In this quiz, you'll test your understanding of Python's namedtuple() function from the collections module. This function allows you to create immutable sequence types that you can access using descriptive field names and dot notation.